/* * Pointers 716 : Card Shuffling And Dealing Modification * * Created on: Feb 29, 2012 * Author: aeriquyairi */ #include<stdio.h> #include<stdlib.h> #include<time.h> void shuffle( int wDeck[][ 13 ] ); void deal( const int wDeck[][ 13 ], const char *wFace[], const char *wSuit[] ); void printDeck( const int wDeck[][ 13 ] ); int main(){ const char *suit[ 4 ] = { "Hearts", "Diamonds", "Clubs", "Spades" }; const char *face[ 13 ] = { "Ace", "Deuce", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King" }; //initialize deck array int deck[ 4 ][ 13 ] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52 }; srand( time( 0 ) ); printf("Initial Deck\n************\n\n"); printDeck( deck ); shuffle( deck ); printf("Shuffled Deck\n*************\n\n"); printDeck( deck ); printf("Deal\n****\n\n"); deal( deck, face, suit ); system("pause"); return 0; } void shuffle( int wDeck[][ 13 ] ){ //shuffling algorithm improvised int row, column, swapRow, swapColumn, hold; for(row = 0; row < 4; row++){ for(column = 0; column < 13; column++){ do{ swapRow = rand() % 4; swapColumn = rand() % 13; }while(wDeck[ swapRow ][ swapColumn ] == wDeck[ row ][ column ]); //swap each element hold = wDeck[ row ][ column ]; wDeck[ row ][ column ] = wDeck[ swapRow ][ swapColumn ]; wDeck[ swapRow ][ swapColumn ] = hold; } } } void printDeck( const int wDeck[][ 13 ] ){ int row, column; for(row = 0; row < 4; row++){ for(column = 0; column < 13; column++){ printf("%5d", wDeck[ row ][ column ]); } printf("\n"); } printf("\n"); } void deal( const int wDeck[][ 13 ], const char *wFace[], const char *wSuit[] ){ //dealing algorithm improvised int card, row, column, dealt; for(card = 1, dealt = 0; card <= 52; card++, dealt = 0){ for(row = 0; row <= 3; row++){ for(column = 0; column <= 12; column++){ if(wDeck[ row ][ column ] == card){ printf("%5s of %-8s%c", wFace[ column ], wSuit[ row ], card % 2 == 0 ? '\n' : '\t' ); dealt = 1; break; } } if(dealt == 1){ break; } } } }
My C Repository
C How to Program
Sweet thanks to my broski Yusuke Fiz for this Deitel book.
You light up my life dude.
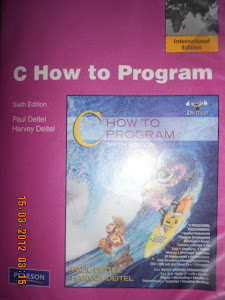
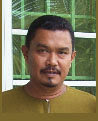
Just another personal public repository.
Friday, March 16, 2012
6) Card Shuffling And Dealing: Modification
The previous shuffling and dealing algorithm is inefficient which may caused the possibility of indefinite postponement.Program below improvised that previous algorithm to avoid indefinite postponement.
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment