/* * Pointers 713 : Evaluate Five Hand Card * * Created on: Feb 23, 2012 * Author: aeriqusyairi */ #include<stdio.h> #include<stdlib.h> #include<time.h> //main functions void shuffle( int wDeck[][ 13 ] ); void deal( const int wDeck[][ 13 ], const char *wFace[], const char *wSuit[] ); void display( const char *wfFace[], const char *wfSuit[], const char *wsFace[], const char *wsSuit[] ); int main(){ //initialize suit array const char *suit[ 4 ] = { "Hearts", "Diamonds", "Clubs", "Spades" }; //initalize face array const char *face[ 13 ] = { "Ace", "Deuce", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King" }; //initialize deck array int deck[ 4 ][ 13 ] = { 0 }; //seed random number generator srand(time(0)); shuffle( deck );//shuffle the deck deal( deck, face, suit );//deal five-hand card system("pause"); return 0; } void shuffle( int wDeck[][ 13 ] ){ int row, column, card; for(card = 1; card <= 52; card++){ do{ row = rand() % 4; column = rand() % 13; }while(wDeck[ row ][ column ] != 0); wDeck[ row ][ column ] = card; } } void deal( const int wDeck[][ 13 ], const char *wFace[], const char *wSuit[] ){ //operational functions void pair( const char *wfFace[], const char *wfSuit[], int result[] ); void twoPair( const char *wfFace[], const char *wfSuit[], int result[] ); void threeKind( const char *wfFace[], const char *wfSuit[], int result[] ); void fourKind( const char *wfFace[], const char *wfSuit[], int result[] ); void flush( const char *wfFace[], const char *wfSuit[], int result[] ); void straight( const char *wfFace[], const char *wfSuit[], const char *wFace[], int result[] ); void eval( const int firstResult[], const int secondResult[] ); int card, row, column, i, j = 0; //storage for two five-hand card //result boolean: 0:pair 1:two pair 2:three of a kind 3:four of a kind 4:flush 5:straight //first char *fSuit[ 5 ] ;//= { "Spades", "Hearts", "Hearts", "Hearts", "Hearts" }; char *fFace[ 5 ] ;//= { "Ace", "Ace", "Three", "Four", "Five" }; int fResult[ 6 ] = { 0 }; //second char *sSuit[ 5 ] ;//= { "Spades", "Hearts", "Hearts", "Hearts", "Hearts" }; char *sFace[ 5 ] ;//= { "Ace", "Six", "Three", "Four", "Five" }; int sResult[ 6 ] = { 0 }; //deal 5-card poker hand for(card = 1, i = 0; card <= 10; card++, i++){ for(row = 0; row < 4; row++){ for(column = 0; column < 13; column++){ if(wDeck[ row ][ column ] == card && i < 5){ fSuit[ i ] = wSuit[ row ]; fFace[ i ] = wFace[ column ]; }else if(wDeck[ row ][ column ] == card && i > 4){ sSuit[ j ] = wSuit[ row ]; sFace[ j ] = wFace[ column ]; j++; } } } } //display two five-card poker hand display( fFace, fSuit, sFace, sSuit ); //evaluate printf("\n******************\nEvaluate both hand\n******************\n\nFirst hand\n**********\n\n"); pair( fFace, fSuit, fResult ); //printf("\n fResult[ 0 ] = %d\n", fResult[ 0 ] ); twoPair( fFace, fSuit, fResult ); //printf("\n fResult[ 1 ] = %d\n", fResult[ 1 ] ); threeKind( fFace, fSuit, fResult ); //printf("\n fResult[ 2 ] = %d\n", fResult[ 2 ] ); fourKind( fFace, fSuit, fResult ); //printf("\n fresult[ 3 ] = %d\n", fResult[ 3 ] ); flush( fFace, fSuit, fResult ); //printf("\n fResult[ 4 ] = %d\n", fResult[ 4 ] ); straight( fFace, fSuit, wFace, fResult ); //printf("\n fResult[ 5 ] = %d\n", fResult[ 5 ] ); printf("\nSecond hand\n***********\n\n"); pair( sFace, sSuit, sResult ); printf("\n sResult[ 0 ] = %d\n", sResult[ 0 ] ); twoPair( sFace, sSuit, sResult ); printf("\n sResult[ 1 ] = %d\n", sResult[ 1 ] ); threeKind( sFace, sSuit, sResult ); printf("\n sResult[ 2 ] = %d\n", sResult[ 2 ] ); fourKind( sFace, sSuit, sResult ); printf("\n sResult[ 3 ] = %d\n", sResult[ 3 ] ); flush( sFace, sSuit, sResult ); printf("\n sResult[ 4 ] = %d\n", sResult[ 4 ] ); straight( sFace, sSuit, wFace, sResult ); printf("\n sResult[ 5 ] = %d\n", sResult[ 5 ] ); //result eval( fResult, sResult ); } void display( const char *wfFace[], const char *wfSuit[], const char *wsFace[], const char *wsSuit[] ){ int i; printf("*************************\nYour five-card poker hand\n*************************\n\nFirst hand %28s\n********** %28s\n\n", "Second hand", "***********"); for(i = 0; i < 5; i++){ printf("%10s of %-8s %10s of %-8s\n", wfFace[ i ], wfSuit[ i ], wsFace[ i ], wsSuit[ i ] ); } } void pair( const char *wfFace[], const char *wfSuit[], int result[] ){ int card, i, j = 0, k; int pair[ 5 ] = { 0 }; //compute whether the five-hand card contains a pair printf("\nPair...\n"); for(card = 0; card < 5; card++){ for(i = card + 1; i < 5; i++){ if(wfFace[ card ] == wfFace[ i ]){ j++; pair[ card ] = i; } } } //display result if(j == 1){ for(card = 0; card < 5; card++){ if(pair[ card ] != 0){ k = pair[ card ]; printf("\n%10s of %-8s and %5s of %-8s\n", wfFace[ card ], wfSuit[ card ], wfFace[ k ], wfSuit[ k ] ); result[ 0 ] = 1; } } }else{ printf("\n Your five-hand card doesn't contains a pair.\n"); } } void twoPair( const char *wfFace[], const char *wfSuit[], int result[] ){ int card, i, j = 0, k; int pair[ 5 ] = { 0 }; //compute whether the five-hand card contains two pair printf("\nTwo-pair...\n"); for(card = 0; card < 5; card++){ for(i = card + 1; i < 5; i++){ if(wfFace[ card ] == wfFace[ i ]){ j++; pair[ card ] = i; } } } //display result if(j == 2){ result[ 1 ] = 1; for(card = 0; card < 5; card++){ if(pair[ card ] != 0){ k = pair[ card ]; printf("\n%10s of %-8s and %5s of %-8s\n", wfFace[ card ], wfSuit[ card ], wfFace[ k ], wfSuit[ k ] ); } } }else{ printf("\n Your five-hand card doesn't contains two pair.\n"); } } void threeKind( const char *wfFace[], const char *wfSuit[], int result[] ){ int card, i, j = 0, k, x; int pair[ 3 ] = { 0 }; //compute whether the five-hand card contains three of a kind printf("\nThree of a kind...\n"); for(card = 0; card < 3; card++){ for(i = card + 1; i < 5; i++){ if(wfFace[ card ] == wfFace[ i ]){ for(x = i + 1; x < 5; x++){ if(wfFace[ i ] == wfFace[ x ]){ j++; pair[ 0 ] = card; pair[ 1 ] = i; pair[ 2 ] = x; } } } } } //display result if(j == 1){ result[ 2 ] = 1; for(card = 0; card < 3; card++){ k = pair[ card ]; printf("\n%10s of %-8s\n", wfFace[ k ], wfSuit[ k ] ); } }else{ printf("\n Your five-hand card doesn't contains three of a kind.\n"); } } void fourKind( const char *wfFace[], const char *wfSuit[], int result[] ){ int card, i, indi = 0, pair[ 5 ] = { 0 }; //compute whether your five-hand card contains four-of-a-kind printf("\nFour of a kind...\n"); for(card = 0; card < 2; card++){ for(i = card + 1; i < 5; i++){ if(wfFace[ card ] == wfFace[ i ]){ indi++; if(indi == 1) pair[ i - 1 ] = 1; pair[ i ] = 1; } } if(indi == 3) break; else if(indi > 3) break; else{ indi = 0; for(i = 0; i < 5; i++) pair[ i ] = 0; } } //display result if(indi == 3){ result[ 3 ] = 1; for(i = 0; i < 5; i++){ if( pair[ i ] == 1) printf("\n%5s of %-9s\n", wfFace[ i ], wfSuit[ i ] ); } printf("\n"); }else{ printf("\n Your five-hand card doesn't contains four-of-a-kind.\n"); } } void flush( const char *wfFace[], const char *wfSuit[], int result[] ){ int i, j = 0; //compute whether your five-hand card contains a flush printf("\nFlush...\n"); for(i = 1; i < 5; i++){ if(wfSuit[ 0 ] == wfSuit[ i ] ) j++; } if(j == 4){ result[ 4 ] = 1; for(i = 0; i < 5; i++){ printf("\n %5s of %-8s\n", wfFace[ i ], wfSuit[ i ] ); } }else{ printf("\n Not flush!\n"); } } void straight( const char *wfFace[], const char *wfSuit[], const char *wFace[], int result[] ){ int i, j, k, pass, count, hold, check = 1; int faceValue[ 5 ] = { 0 }; printf("\nStraight...\n"); //locate face value and store in an array for(i = 0 ; i < 5; i++){ for(j = 0 ; j < 13; j++){ if(wfFace[ i ] == wFace[ j ]){ faceValue[ i ] = j; } } } //sort face value in ascending order using bubble sort for(pass = 0; pass < 4; pass++){ for(count = 0; count < 4; count++){ if(faceValue[ count ] > faceValue[ count + 1 ]){ //swap hold = faceValue[ count ]; faceValue[ count ] = faceValue[ count + 1 ]; faceValue[ count + 1 ] = hold; } } } //check if the hand contains a straight for(i = 0; i < 4; i++){ if(faceValue[ i ] + 1 != faceValue[ i + 1 ]){ check = 0; } } if(check == 1){ result[ 5 ] = 1; for(i = 0; i < 5; i++){ printf("\n %5s of %-8s\n", wfFace[ i ], wfSuit[ i ] ); } }else{ printf("\n Not a straight hand.\n" ); } } void eval( const int firstResult[], const int secondResult[] ){ int i, x = 0, y = 0; printf("\n******\nResult\n******\n\n"); //debug /* for(i = 0; i < 6; i++){ int j = 0; if(firstResult[ i ] == 1 && secondResult[ i ] == 1){ printf("%d = Its a tie!\n", i ); j = 1; }else if(firstResult[ i ] == 1){ printf("%d = First hand is better.\n", i ); j = 1; }else if(secondResult[ i ] == 1){ printf("%d = Second hand is better.\n", i ); j = 1; }else printf("%d = Both hand's weak!\n", i ); } printf("\n"); */ //evaluate both hand for(i = 0; i < 6; i++){ if(firstResult[ i ] == 1) x = i + 1; if( secondResult[ i ] == 1) y = i + 1; } //debug printf("x = %d y = %5d\n\n", x, y); if(x > y) printf("First hand win!\n\n"); else if(y > x) printf("Second hand win!\n\n"); else if(x == y) printf("Its a tie!\n\n"); else printf("Unable to evaluate!\n\n"); }
My C Repository
C How to Program
Sweet thanks to my broski Yusuke Fiz for this Deitel book.
You light up my life dude.
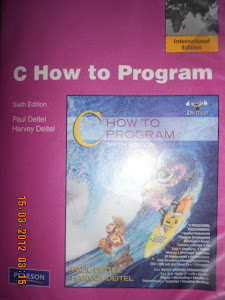
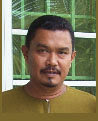
Just another personal public repository.
Friday, March 16, 2012
3) Card Shuffling and Dealing: Five-card Poker Hand Evaluation
Deals two five-card poker hand, evaluates each hand, and determines which is the better hand.
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment